Code coverage is a crucial metric in software development, particularly in Java, as it helps ensure that software is well-tested and reliable. It measures how much of the codebase is executed during testing, providing valuable insights into the effectiveness of tests. High code coverage indicates that most of the code has been tested, reducing the chances of undetected bugs.
Understanding Code Coverage
Code coverage is expressed as a percentage, representing the proportion of code executed during testing. It is especially useful in unit testing and test automation, where developers need to gauge the thoroughness of their test cases.
A well-tested application is less likely to contain hidden defects, increasing stability and maintainability. While 100% code coverage does not guarantee a bug-free application, it does show that tests have executed all lines, branches, or paths in the code.
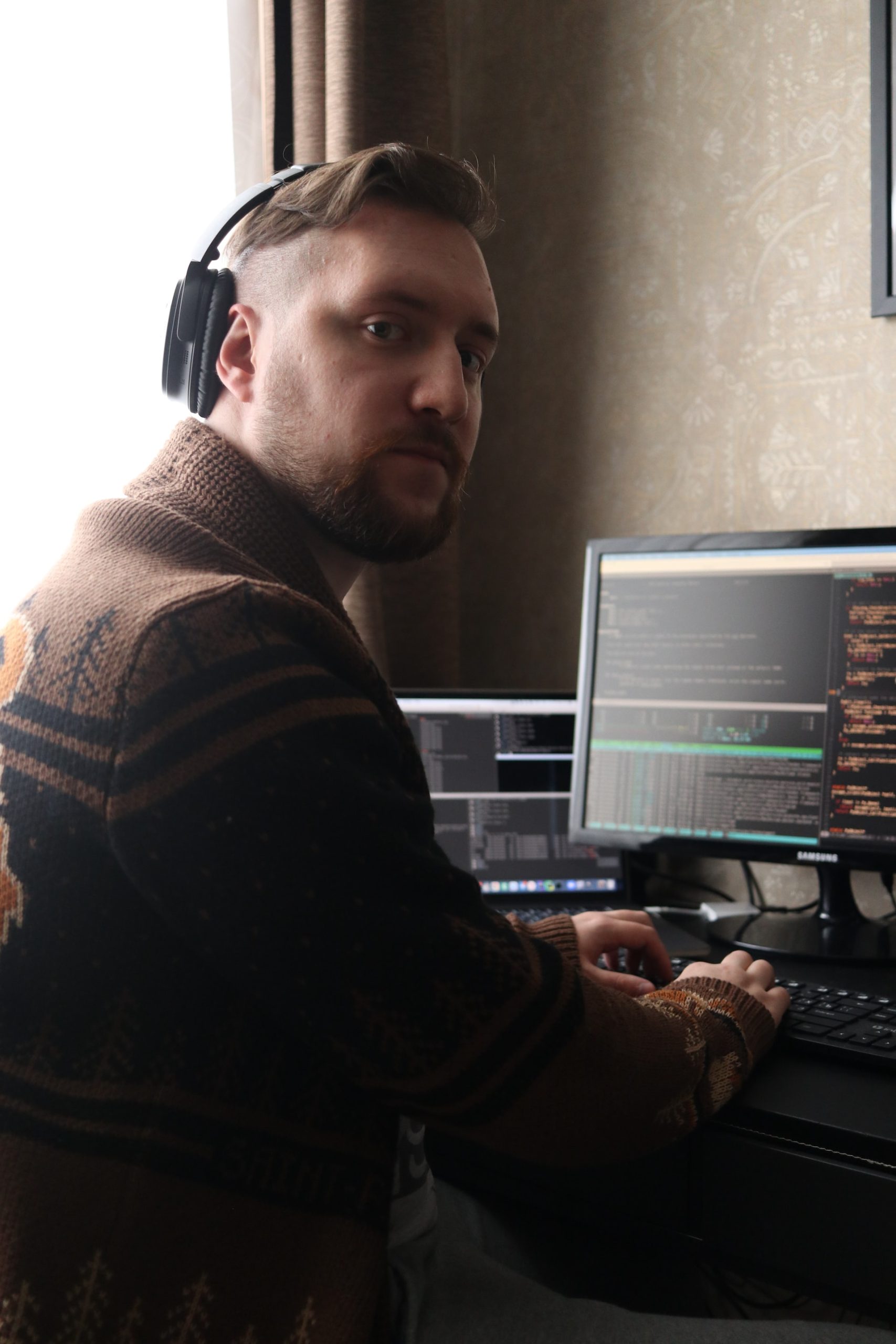
Types of Code Coverage
There are several types of code coverage, each emphasizing different aspects of the code execution:
- Statement Coverage: Measures the percentage of program statements executed at least once during testing.
- Branch Coverage: Ensures that every decision point (such as if-else conditions) is covered in all possible outcomes.
- Path Coverage: Examines the execution flow of all possible paths through the codebase.
- Function Coverage: Checks whether all defined functions or methods are executed.
- Condition Coverage: Ensures that each Boolean sub-expression in the code evaluates to both true and false.
Each type of coverage offers unique benefits, making it essential to use more than one approach for thorough test validation.
How to Measure Code Coverage in Java
Java developers can measure code coverage using several tools designed to analyze test execution and report coverage statistics. Some of the most popular tools include:
- Jacoco: A widely-used coverage tool integrated with builds using Maven or Gradle.
- Emma: A flexible tool designed for Java applications, providing detailed coverage reports.
- Cobertura: An open-source coverage tool that generates detailed reports for Java code.
- JUnit and Clover: Tools commonly combined with unit testing frameworks such as JUnit.
These tools provide reports that help developers identify untested parts of the codebase, allowing them to improve test coverage.
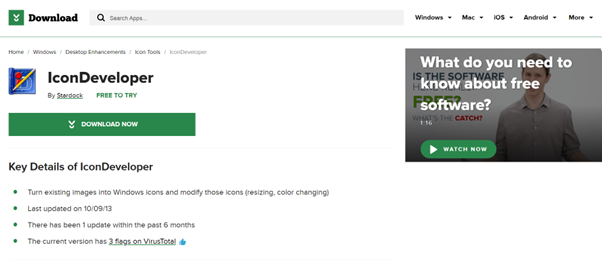
Best Practices for Achieving High Code Coverage
To ensure high-quality and reliable code, developers should follow these best practices to maximize code coverage:
- Write Comprehensive Unit Tests: Test all critical functions and edge cases to prevent potential issues.
- Use Mocking and Stubbing: Isolate the code you are testing using mocking frameworks like Mockito.
- Analyze Coverage Reports: Identify untested areas and improve test cases based on report insights.
- Automate Testing Processes: Integrate testing into Continuous Integration (CI) pipelines to ensure consistent execution.
- Aim for Meaningful Coverage: Rather than merely chasing higher percentages, focus on testing essential and complex logic.
Limitations of Code Coverage
While code coverage is an important metric, it does not guarantee software quality. Here are some common limitations:
- Missed Edge Cases: Even with high coverage, tests may not cover all possible input conditions.
- Lack of Functional Testing: Code coverage focuses on whether code executes but not necessarily on whether it works as expected.
- False Sense of Security: A high coverage percentage can be misleading if tests do not validate actual behavior.
For these reasons, code coverage should be used alongside other quality assurance techniques, such as functional testing and code reviews.
Conclusion
Code coverage plays a pivotal role in ensuring well-tested, maintainable, and reliable Java applications. By leveraging modern tools and best practices, developers can enhance their testing strategies and produce higher-quality software. However, it is essential to remember that achieving high code coverage is only part of the overall software quality assurance process. A balanced approach that includes unit testing, functional testing, and manual review leads to robust and dependable applications.