When using PHP Selenium WebDriver to automate browser interactions, developers sometimes encounter unexpected redirections when calling the sendKeys
method. This issue can lead to unintended navigation away from the intended page and disrupt test execution. Understanding why this happens and how to prevent it is crucial for maintaining reliable automation scripts.
Understanding the sendKeys
Method
In Selenium, the sendKeys
method simulates typing into an input field or interacting with elements by sending keystrokes. Normally, this method should only enter text into an input field without causing redirections. However, certain scenarios can trigger page navigation unexpectedly, leading to unreliable test results.
Common Causes of Redirects
Several factors might contribute to unintended redirections when using sendKeys
in PHP Selenium WebDriver:
- JavaScript Event Listeners: Some web applications use JavaScript event listeners that trigger page reloads or redirects when text is entered into an input field.
- Form Auto-Submission: Some forms are configured to auto-submit when text is added to an input field, especially when pressing โEnterโ is simulated.
- Field Autocomplete Behavior: Certain sites implement autocomplete features that change the pageโs state upon text entry.
- Misconfigured WebDriver Interaction: Some browsers or Selenium versions may interpret
sendKeys
as triggering a form submission depending on the elementโs behavior.
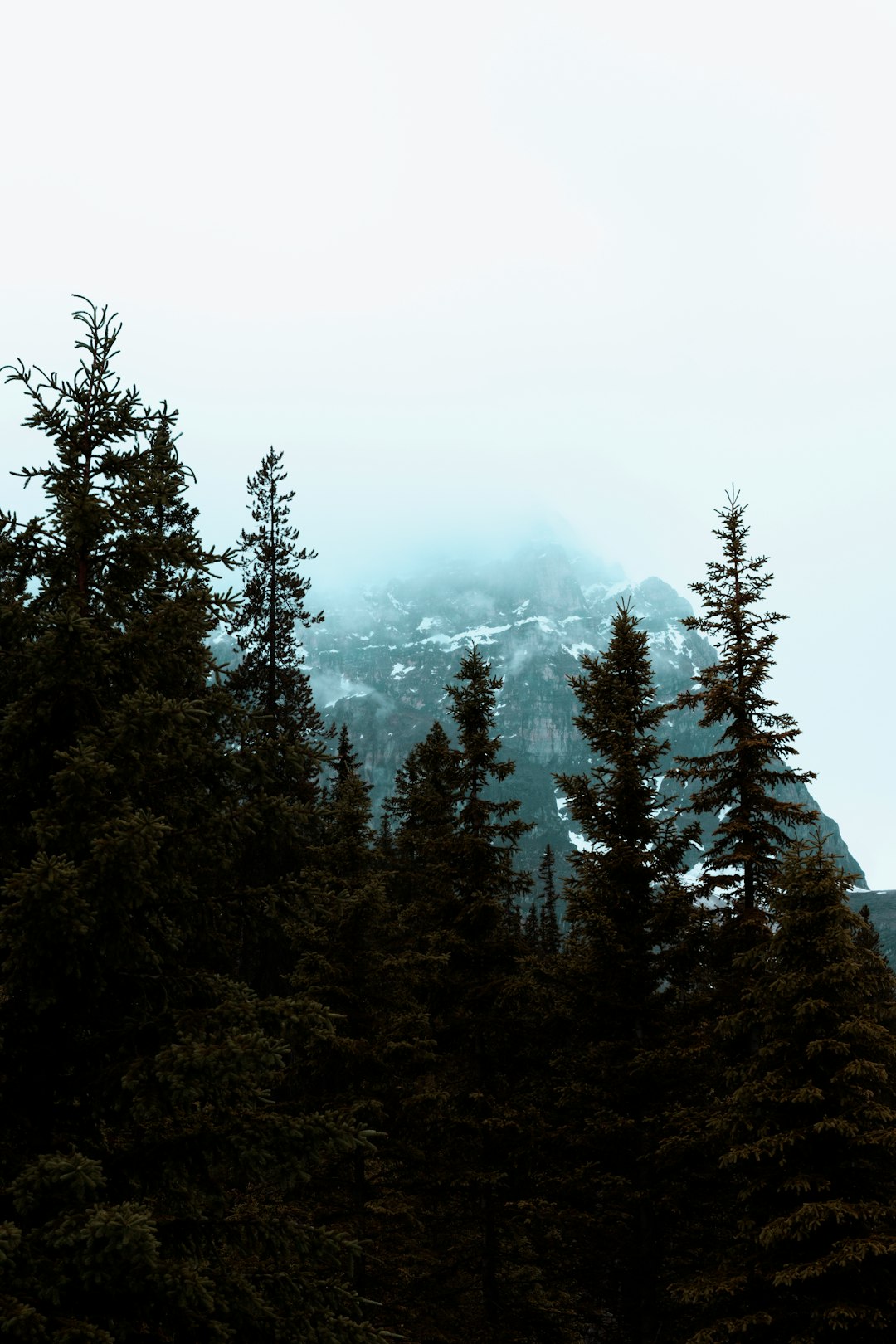
How to Prevent Unexpected Redirects
To avoid unintended page navigation when using sendKeys
, try the following solutions:
1. Use JavaScript to Set the Value
Instead of sending keys through Selenium, set the input fieldโs value using JavaScript. This method bypasses event listeners that might trigger a redirect.
$driver->executeScript("arguments[0].value = 'Test Input';", [$element]);
2. Send Keys Without Pressing Enter
Ensure that no unintended โEnterโ keypress is sent along with the input text. If youโre using sendKeys
, provide only the necessary text without appending \n
:
$element->sendKeys('Test Input');
3. Wait for Input Processing
In some cases, adding a short delay after entering text can help prevent automatic submission.
$element->sendKeys('Test Input');
sleep(1);
Though not always the best solution, this can be useful when debugging the cause of redirections.
4. Disable JavaScript for Testing
Disabling JavaScript temporarily can help determine whether the redirection is caused by client-side scripts. This can be done by configuring Selenium WebDriver to disable JavaScript execution:
$capabilities = new DesiredCapabilities();
$capabilities->setCapability('javascriptEnabled', false);
$driver = RemoteWebDriver::create($seleniumServerUrl, $capabilities);
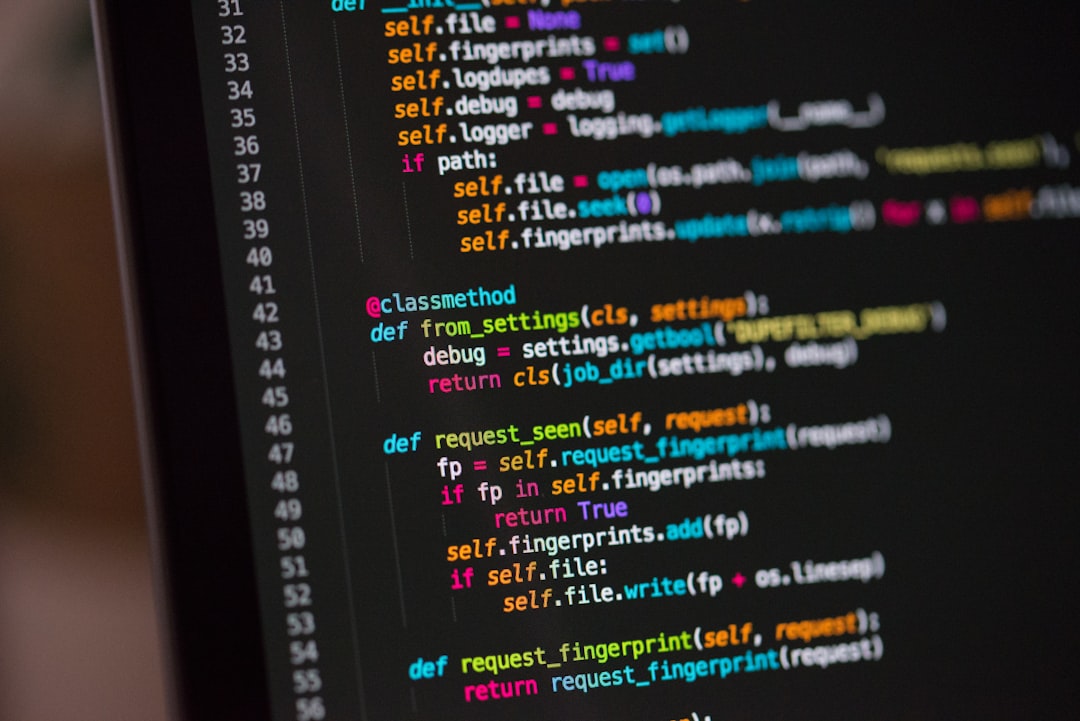
Using ActionChains
for More Control
Sometimes, using sendKeys
alone induces unexpected behavior. To have finer control over form interactions, use Actions
:
$actions = new \Facebook\WebDriver\Interactions\WebDriverActions($driver);
$actions->moveToElement($element)->click()->sendKeys('Test Input')->perform();
This ensures that focus and text input behavior simulate a real user interaction more effectively.
Conclusion
Unexpected redirects when using sendKeys
in PHP Selenium WebDriver can disrupt automated tests and cause unreliable behavior. By understanding the possible causes, such as JavaScript event listeners, auto-submission, and improper handling of input fields, developers can implement appropriate solutions to mitigate these issues.
Using JavaScript for direct value setting, controlling keystroke behavior, and leveraging WebDriver Actions are effective approaches. Debugging the redirection cause by disabling JavaScript or adding delays can also provide useful insights. By applying these best practices, developers can ensure smoother and more predictable test execution.