Drawing a rectangle, setting its border width, and filling it with color is a fundamental aspect of graphics programming. Whether you are working with HTML5 Canvas, SVG, or CSS, understanding how to achieve this effect will help you create visually appealing designs and user interfaces. In this article, we will explore different ways to draw a rectangle, customize its appearance, and use colors effectively.
Using HTML5 Canvas
The HTML5 <canvas>
element provides a powerful way to create 2D graphics using JavaScript. To draw a rectangle and style it properly, follow these steps:
- Create a
<canvas>
element in your HTML. - Access the canvas using JavaScript and obtain the drawing context.
- Draw your rectangle using the
fillRect()
method. - Set the border width and stroke color using
lineWidth
andstrokeStyle
. - Use the
fillStyle
property to define the fill color.
Hereβs a simple example:
<canvas id="myCanvas" width="200" height="150"></canvas>
<script>
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
// Set fill color
ctx.fillStyle = "lightblue";
ctx.fillRect(20, 20, 150, 100);
// Set border width and color
ctx.lineWidth = 5;
ctx.strokeStyle = "black";
ctx.strokeRect(20, 20, 150, 100);
</script>
This will create a light blue rectangle with a black border that is 5 pixels wide.
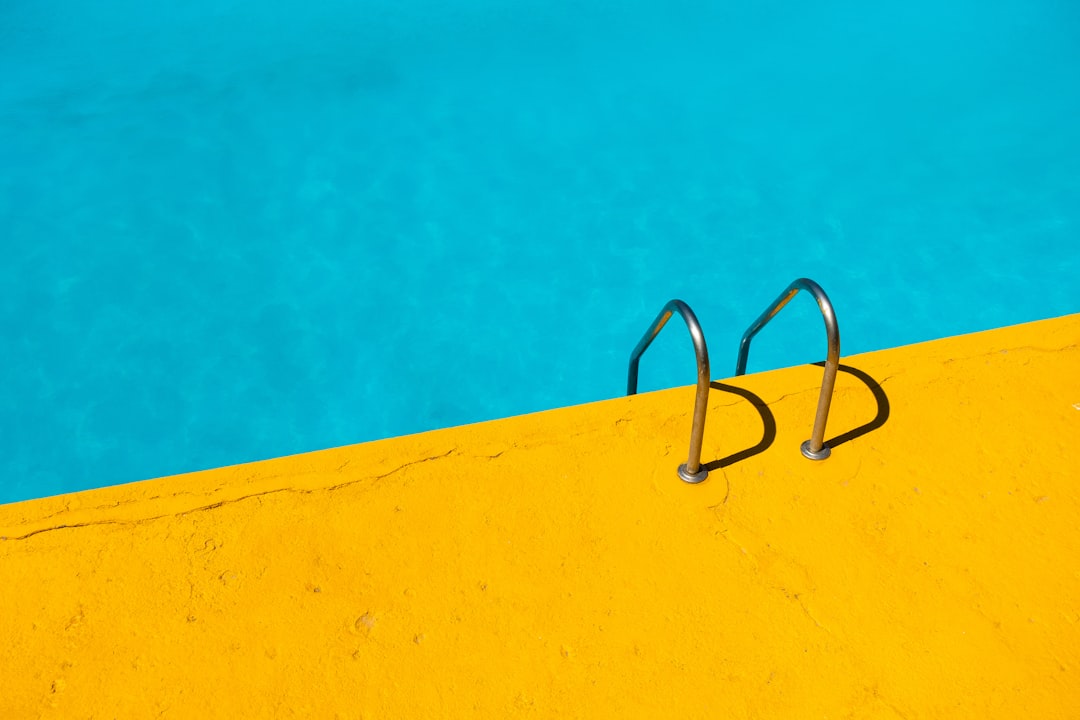
Using SVG
SVG (Scalable Vector Graphics) is another way to create rectangles in web design. It allows for high-quality rendering without losing resolution. Hereβs how you can create an SVG rectangle:
<svg width="200" height="150">
<rect x="20" y="20" width="150" height="100"
fill="lightblue" stroke="black" stroke-width="5"/>
</svg>
In this example:
x
andy
define the rectangleβs position.width
andheight
determine its dimensions.fill
sets the fill color.stroke
andstroke-width
define the border color and width.
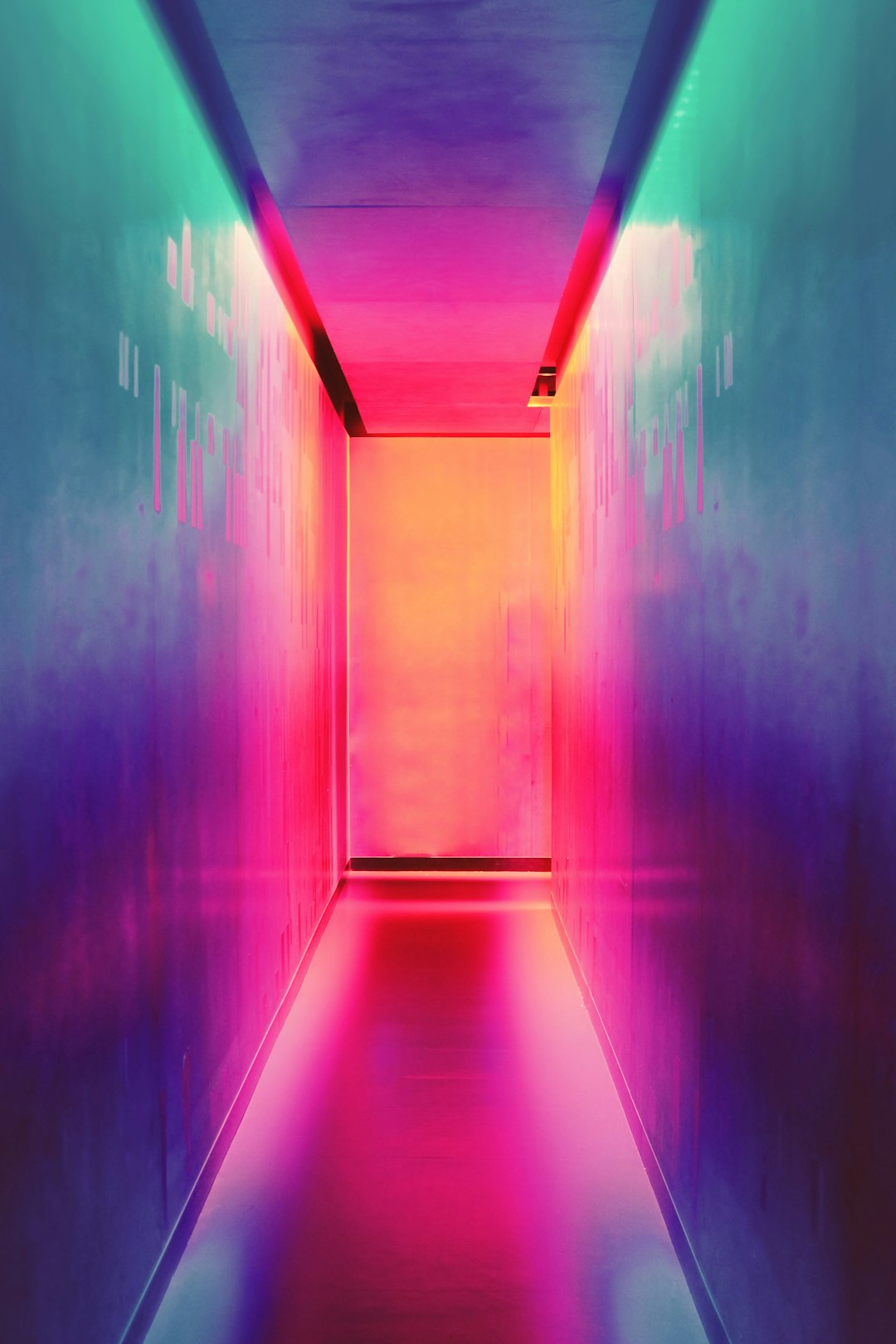
Using CSS
For simple rectangles with background colors and border styling, CSS is a great option. You can use the <div>
element and apply styles:
<div class="rectangle"></div>
<style>
.rectangle {
width: 150px;
height: 100px;
background-color: lightblue;
border: 5px solid black;
}
</style>
With CSS, you can also apply additional styles such as rounded corners, shadows, and animations to make your rectangles more visually appealing.
Choosing the Right Method
The method you choose depends on your needs:
- Use HTML5 Canvas if you need dynamic drawing with JavaScript.
- Use SVG if you require scalable graphics with vector support.
- Use CSS for simple design elements without extra JavaScript.
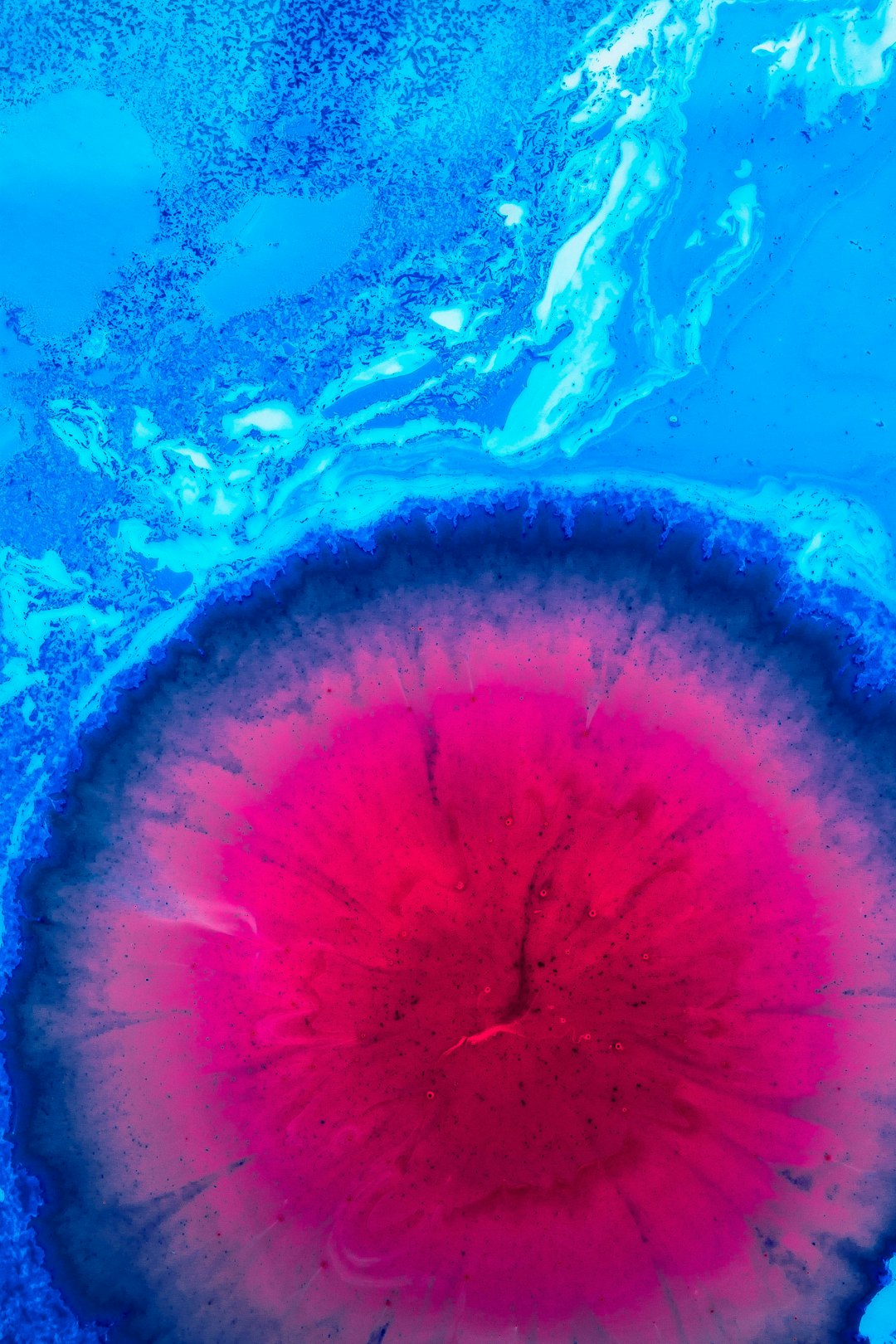
Conclusion
Drawing rectangles, setting borders, and filling them with color is an essential skill in web design and graphics programming. Whether youβre using HTML5 Canvas, SVG, or CSS, understanding how to manipulate these properties correctly will help you create stunning visuals in your projects.